Including telephone number in short links
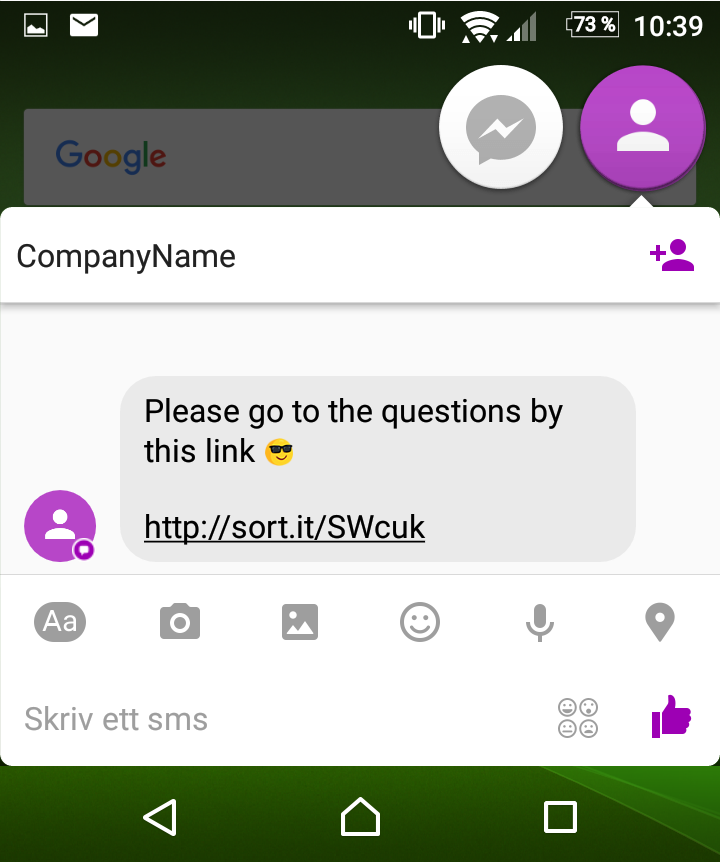
Sending SMS links is very common, and it is often interesting to know who clicked the link in the SMS. Since the web browser does not know what phone it is running on - a unique ID is needed for each SMS. It is possible to use an URL shortner like (goo.gl) that makes links like this http://goo.gl/s6zIe2 .
URL-Shortener limitations
However using URL-Shorteners has some limitations:
- Privacy is limited since lots of free solutions publish your links.
- Speed is limited since your need to make an API request for each phone number.
- Number of possible urls are limited (due to limits in the shortner service).
- Cost to avoid the problems of Privacy, Speed and amount limits you may pay for services, however this is often quite expensive.
Encode number as short string
To solve this issues and still keep the number information - you may encode the number as an encoded string. This way a number like 766861004 can be coded as "SWcuk" and put in an example url like: http://sortu.rl/SWcuk
"SWcuk" can then be decoded to the original number with a simple self contained function. No access to a database is needed.
Benefits
- No limit in number of urls created.
- Central database for translation urls is not needed.
- Low cost.
- Number link connection is keep all the way in the flow.
Downsides
- Only works for numbers.
- Not possible to use for general URLs.
Example of implementation in python
alphabet = "0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz_-~=+/.!&"
charscount = len(alphabet)
def encode(n):
n = int(n)
encoded = ''
while n > 0:
n, r = divmod(n, charscount)
encoded = alphabet[r] + encoded
return encoded
def decode(s):
decoded = 0
while len(s) > 0:
decoded = decoded * charscount + alphabet.find(s[0])
s = s[1:]
return str(decoded)
Example of implementation in PHP
function encode($number){
$alphabet = "0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz_-~=+/.!&";
$charscount = strlen($alphabet);
$number = intval($number);
while($number > 0){
$reminder = $number % $charscount;
$number = intval($number/$charscount);
$encoded = substr($alphabet,$reminder,1).$encoded;
}
return $encoded;
}
function decode($string){
$alphabet = "0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz_-~=+/.!&";
$charscount = strlen($alphabet);
$number = 0;
while(strlen($string) > 0){
$number = $number * $charscount + strpos($alphabet,substr($string,0,1));
$string = substr($string,1);
}
return $number;
}
Hashids generate short unique ids from integers in a similar way.