What you'll need
- Join the 46elks Slack workspace or the Slack space of your choice ofc ππΌ
- Create a 46elks account
- A virtual number We have set you up with a Swedish phone number on your account that you can use for free during one year
- A live server to run your code on. Text your first name to +46766865445 to get an account on our live workshop server. You'll need this SMS for the next steps β¬οΈ
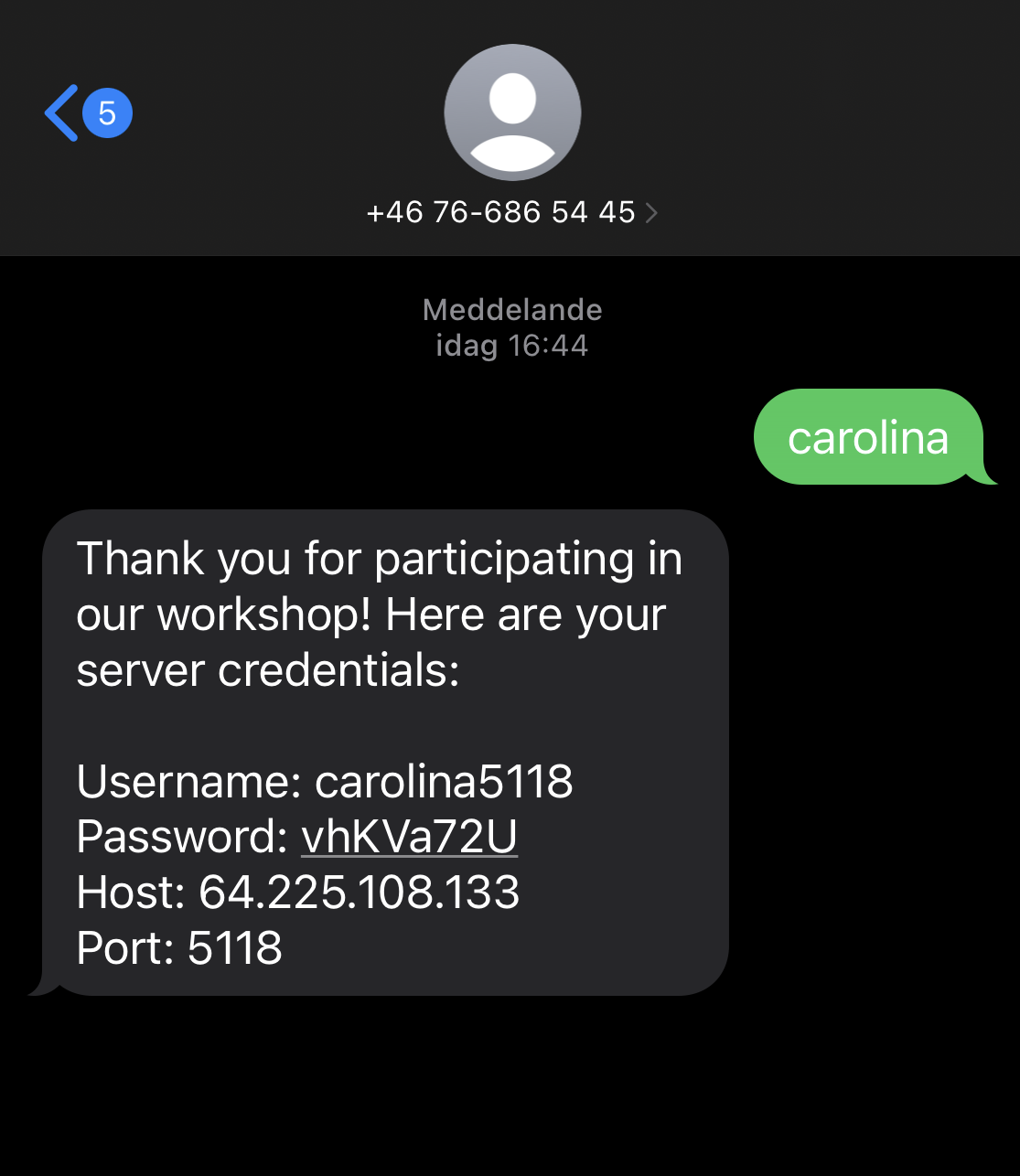
What we're doing today
Be patient with yourself and others. We'll help each other out!
If you need help during the workshop, just write in the chat or ask one of us for help and we'll fix it together ππΌ
- Access the live server
- Set up Python & Flask
- Learn to send SMS with Python on a live server
- Set up a Slack webhook
- Modify your virtual number
- And if it all goes right: Receive SMS in Slack π
Send SMS with Python
Log in to the server
- Log in to the server with the credentials that you got in the SMS
- Swap
username
to your username in the SMS you got from the workshop server - Use the password you got and you're in
Send a SMS
- Create your first python file here with
nano send-sms.py
- Copy and paste this in to your new file
CTRL+X
and thenY
to save your file- To run your Python script on the server now you run
python send-sms.py
in your Terminal/Command line - If everything worked you should have gotten a
Response [200]
response
Terminology to know about APIs
Now you have sent your first SMS with Python - Quick and easy as that! π²
There are a couple of words that you'll want to know about when working with APIs, it will make it easier for you to troubleshoot and find help.
- url
- request
- response
- http status codes
- docs
- GET & POST
- Authentication
Set up Slack app and webhook
- Join our 46elks Slack workspace or create your own
- Create a slack app
- In your Slack workspace, go to the "Apps" section and create a new Slack app.
- Give your app a name and associate it with the right workspace for example 46elks.
- Add a webhook integration in your app
- Inside your Slack app settings, navigate to the "Incoming Webhooks" section.
- Activate the Incoming Webhooks feature if it's not already enabled.
- Click on "Add New Webhook to Workspace."
- Choose a channel to post your incoming SMS in
- Select the channel where you want to send the SMS messages. You can choose an existing channel or create a new one.
- Click "Allow" to authorize your app to post messages to the selected channel.
- Copy your webhook URL and store in a smart place, we are going to use that later
Set up a Flask app and our Python code
- Let's set up Flask to create our virtual enviroment. Run each command in your command line.
python3 -m venv slack_sms
this creates a isolated Python enviroment named "slack_sms". Used to manage project specific dependencies.. slack_sms/bin/activate
activates the enviroment. To stop the enviroment you run:deactivate
cd slack_sms
to enter the env you just created and activatedpip install requests
installing the Python library Requests in order to make HTTP requestspip install Flask
installs Flask, a web framework to create your web application- Import Flask and name it by changing the variable name
- At the top of the file,
import requests
- Set up new route. Here we are creating an endpoint at /incoming-sms that listens for HTTP POST requests
- Handle incoming SMS and save it to variables that we'll use later on
- Define our Slack URL that we copied and stored previously
- Create a payload for Slack (what will we forward to Slack)
- Forward the SMS to Slack
- Handle the response to check success or for troubleshooting
- Start your Flask app. Replace
5000
with your own port number
Write the Python code
Create a new python file sms-slack.py
and now let's code our SMS integration
Configure our 46elks number
- Navigate in to 46elks dashboard and click on "Numbers"
- Next to your number you'll click on "Edit"
- Now we set up our sms_url. It's our webhook, it's the server URL + the endpoint that we set up in our app route. Ex like this
http://64.225.108.133:5000/incoming-sms
. Replace5000
to your port number.
Let's see if it works
Start the Flask app
Now we start our Flask app in the command line and see if it works
- Run your Flask app. Replace
5000
with your own port number - To stop Flask run
CTRL+C
- Visit your Flask app. Replace
5000
with your own port number
Now you send a SMS to your virtual number and see if it gets forwarded to your Slack channel - Let's test it and see what happens π
Resources
The full code is here in case something happened to yours on the way